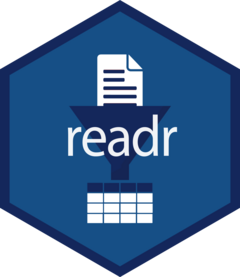
Function reference
Read rectangular files
These functions parse rectangular files (like csv or fixed-width format) into tibbles. They specify the overall structure of the file, and how each line is divided up into fields.
-
read_delim()
read_csv()
read_csv2()
read_tsv()
- Read a delimited file (including CSV and TSV) into a tibble
-
read_fwf()
fwf_empty()
fwf_widths()
fwf_positions()
fwf_cols()
- Read a fixed width file into a tibble
-
read_log()
- Read common/combined log file into a tibble
-
read_table()
- Read whitespace-separated columns into a tibble
Column specification
The column specification describes how each column is parsed from a character vector in to a more specific data type. readr does make an educated guess about the type of each column, but you’ll need override those guesses when it gets them wrong.
-
problems()
stop_for_problems()
- Retrieve parsing problems
-
cols()
cols_only()
- Create column specification
-
cols_condense()
spec()
- Examine the column specifications for a data frame
-
spec_delim()
spec_csv()
spec_csv2()
spec_tsv()
spec_table()
- Generate a column specification
Column parsers
Column parsers define how a single column is parsed, or how to parse a single vector. Each parser comes in two forms: parse_xxx()
which is used to parse vectors that already exist in R and col_xxx()
which is used to parse vectors as they are loaded by a read_xxx()
function.
-
parse_logical()
parse_integer()
parse_double()
parse_character()
col_logical()
col_integer()
col_double()
col_character()
- Parse logicals, integers, and reals
-
parse_datetime()
parse_date()
parse_time()
col_datetime()
col_date()
col_time()
- Parse date/times
-
parse_factor()
col_factor()
- Parse factors
-
parse_guess()
col_guess()
guess_parser()
- Parse using the "best" type
-
parse_number()
col_number()
- Parse numbers, flexibly
-
col_skip()
- Skip a column
Locale controls
The “locale” controls all options that vary from country-to-country or language-to-language. This includes things like the character used as the decimal mark, the names of days of the week, and the encoding. See vignette("locales")
for more details.
-
locale()
default_locale()
- Create locales
-
date_names()
date_names_lang()
date_names_langs()
- Create or retrieve date names
Write rectangular files
Despite its name, readr also provides a number of functions to write data frames to disk, or to convert them to in-memory strings.
-
format_delim()
format_csv()
format_csv2()
format_tsv()
- Convert a data frame to a delimited string
-
write_delim()
write_csv()
write_csv2()
write_excel_csv()
write_excel_csv2()
write_tsv()
- Write a data frame to a delimited file
Readr editions
readr supports two editions of parser. Version one is a single threaded eager parser that readr used by default from its first release to version 1.4.0. Version two is a multi-threaded lazy parser used by default from readr 2.0.0 onwards.
-
with_edition()
local_edition()
- Temporarily change the active readr edition
-
edition_get()
- Retrieve the currently active edition
Read non-rectangular files
These functions parse non-rectangular files (like csv or fixed-width format) into long (so-called melted) format. They specify the overall structure of the file, and how each line is divided up into fields.
-
melt_delim()
melt_csv()
melt_csv2()
melt_tsv()
- Return melted data for each token in a delimited file (including csv & tsv)
-
melt_fwf()
- Return melted data for each token in a fixed width file
-
melt_table()
melt_table2()
- Return melted data for each token in a whitespace-separated file
Low-level IO and debugging tools
These functions can be used with non-rectangular files, binary data, and to help debug rectangular files that fail to parse.
-
read_file()
read_file_raw()
write_file()
- Read/write a complete file
-
read_lines()
read_lines_raw()
write_lines()
- Read/write lines to/from a file
-
read_rds()
write_rds()
- Read/write RDS files.
-
read_builtin()
- Read built-in object from package
-
count_fields()
- Count the number of fields in each line of a file
-
guess_encoding()
- Guess encoding of file
-
type_convert()
- Re-convert character columns in existing data frame
-
readr_example()
- Get path to readr example
-
clipboard()
- Returns values from the clipboard
-
show_progress()
- Determine whether progress bars should be shown
-
readr_threads()
- Determine how many threads readr should use when processing
-
should_show_types()
- Determine whether column types should be shown
-
should_read_lazy()
- Determine whether to read a file lazily